Earlier today, Josh (a fellow intern and blog contributor) wrote a blog post about logic gates. After reading through Josh’s post and gaining an understanding of the concepts and basic functions of those gates, I figured now would be the perfect time to learn some code. I am going to go over each logic gate and it’s code in Verilog (a hardware language), VHDL (another hardware language) and C (software language). If you need a refresher on what a hardware language is, you can read about Verilog vs. VHDL. Each gate is shown below with the code for each language in their respective colors.
NOT:
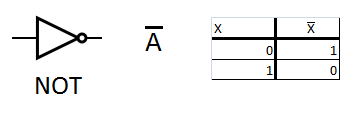
assign Y = ~A;
Y <= NOT A;
Y = !A;
AND:
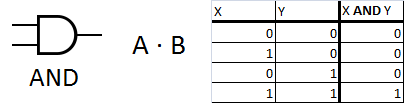
assign Y = A & B;
Y <= A AND B;
Y = A && B;
NAND:
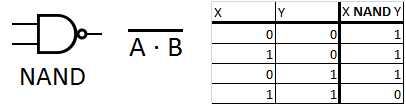
assign Y = ~(A & B);
Y <= A NAND B;
Y = !(A && B);
OR:
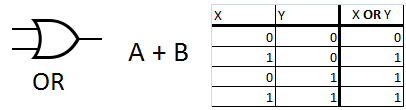
assign Y = A|B;
Y <= A OR B;
Y = A || B;
NOR:
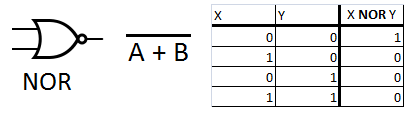
assign Y = ~(A|B);
Y <= A NOR B;
Y = ! (A || B);
XOR:
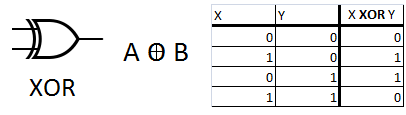
assign Y = A^B;
Y <= A XOR B;
Y = ((!A) && B) || (A && (!B));
XNOR:
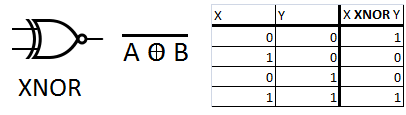
assign Y = A^B;
Y <= A XNOR B;
Y = !((!A) && B) || (A && (!B));
As you can see from the examples of all of these gates, Verilog and VHDL aren’t all that different from C in terms of syntax. In fact, C has bitwise logic operators that are the same as those of the hardware languages. However, bitwise operations aren’t very common in C. Unfortunately, C doesn’t have a symbol for XOR and XNOR, so those had to be made out of AND, OR, and NOT gates.
This content is relevant to the Basys 3, Nexys A7, Cmod A7, and Cmod S7. All can be found on our FPGA product page.
Hi,
please fix the VHDL code lines
Y <= NOT A;
Y <= A AND B;
….
Y is not an assignmet operator in VHDL
Please fix the tables :
A B | A AND B
————————-
….
this will bring table and picture in sync
Hi Martin,
I’m not sure how my brain swapped the less than and greater than signs. That is all fixed now.
As far as the images go I’ll have to work on getting those fixed, as they are borrowed from another post.
Thanks for your feedback!